🏦 How I built an open source hedgefund
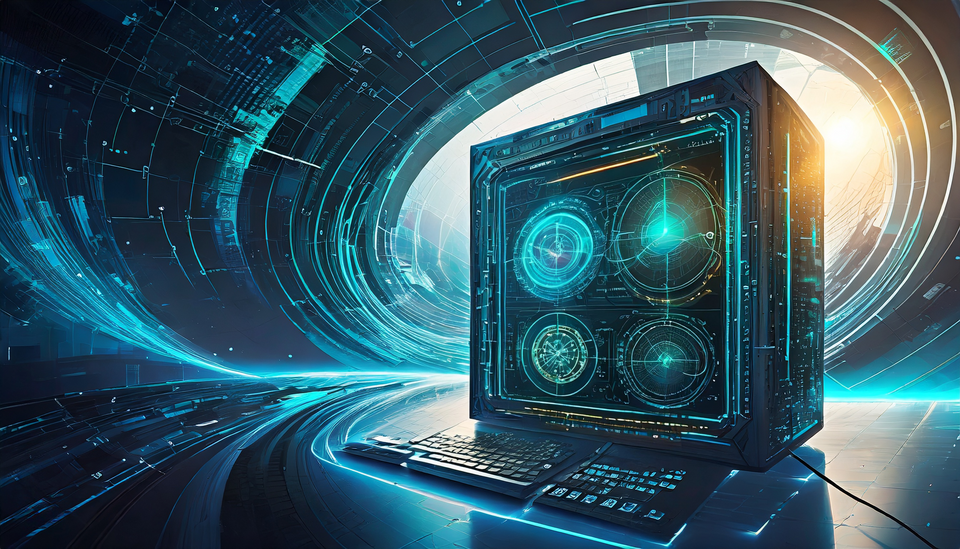
Earlier this year I built out a lightweight python API to access Coinbase's new trading platform Advanced Trade
Coinbase is the most used cryptocurrency platform in the US and I wanted to make the Gemini API tools from the channel available to a wider audience
The first trading strategy I've built out and released to users of the API is the fear and greed index
(I explain the fear and greed index and do some backtesting here if you want to learn more)
How to use the API
To get started with the API install the pip package using this terminal command
pip install coinbase-advancedtrade-python
Or download this layer.zip for use in AWS (if you want to generate your own layer follow these instructions)
Connecting to Coinbase
from coinbase_advanced_trader.config import set_api_credentials
# Set your API key and secret
API_KEY = "ABCD1234"
API_SECRET = "XYZ9876"
# Set the API credentials once, and it updates the CBAuth singleton instance
set_api_credentials(API_KEY, API_SECRET)
Replace the placeholder values for API_KEY and API_SECRET with your Coinbase account API keys
You can generate API keys by going to Coinbase.com -> Profile Picture -> Settings -> API -> New API Key.
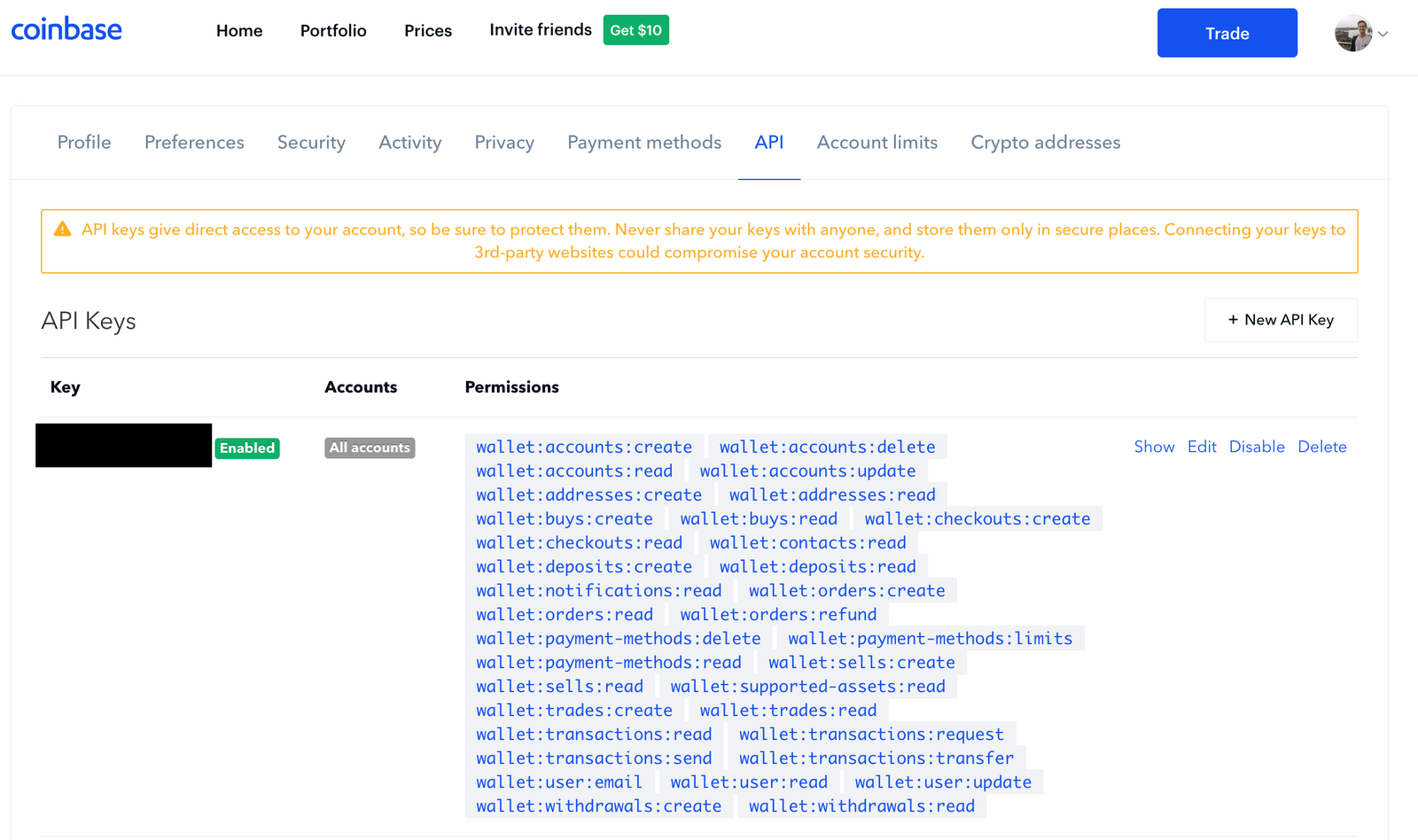
Fear and Greed Index
Fear and Greed trades work by defining three variables
1) The threshold value of the Fear and Greed Index
2) A factor to change your trade size
3) An action to take (buy or sell)
In most strategies you would: increase your buy size if the Fear/Greed value was low and decrease your buy size or increase your sell size if the Fear/Greed value was high
Here are some example schedules:
{"threshold": 20, "factor": 1, "action": "buy"},
{"threshold": 80, "factor": 0.5, "action": "buy"},
{"threshold": 100, "factor": 1, "action": "sell"}
Buys 1x when fear and greed is less than 20
Buys .5x when fear and greed is more than 20 and less than 80
Sells 1x when fear and greed is more than 80 and less than 100
{"threshold": 40, "factor": 1.5, "action": "buy"},
{"threshold": 60, "factor": .75, "action": "buy"},
{"threshold": 80, "factor":.25, "action": "buy"}
Buys 1.5x when fear and greed is less than 40
Buys .75x when fear and greed is more than 40 and less than 60
Buys .25x when fear and greed is more than 60 and less than 80
Does nothing when fear and greed is more than 80
You can set your own custom schedule to satisfy whatever your trading strategy calls for
Code Examples
For very simple fear and greed use cases you can use trade_based_on_fgi_simple
from coinbase_advanced_trader.strategies.fear_and_greed_strategies import trade_based_on_fgi_simple
# Define the product id
product_id = "BTC-USD"
# Implement the strategy
trade_based_on_fgi_simple(product_id, 10)
The code above:
If Fear and Greed < 20: Buys $12 of Bitcoin
If Fear and Greed <80 and >20: Buys $10 of Bitcoin
If Fear and Greed > 80: Sells $8 of Bitcoin
For more complicated use cases you can utilize the Pro function and define your own schedule:
from coinbase_advanced_trader.strategies.fear_and_greed_strategies import trade_based_on_fgi_pro
# Define the product id
product_id = "BTC-USD"
# Define the custom schedule
custom_schedule = [
{"threshold": 20, "factor": 1, "action": "buy"},
{"threshold": 80, "factor": 0.5, "action": "buy"},
{"threshold": 100, "factor": 1, "action": "sell"},
]
# Implement the strategy
response = trade_based_on_fgi_pro(product_id, 10, custom_schedule)
Customizing your script
If you're not a great programmer and you want to build something yourself it's never been easier thanks to ChatGPT and Cursor.sh
Full Cursor.sh tutorial here
Simply download the code from git, open it in Cursor.sh and ask Cursor.sh to build you a test file using @codebase that does whatever you want it to do
What's next?
The coolest project I've seen so far forking from this repository is a telegram trading bot built by Thomaslc66
The possibilities here are endless so feel free to submit pull requests, email feature requests (rhett@rhett.blog) or dm me feedback over on Twitter
Or fork the repository yourself and build something cool like Thomas did.
I'll try to get new strategies incorporated so that this codebase can deliver even more trading strategies.